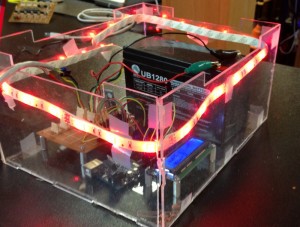
This control box receives commands wirelessly via bluetooth and sends commands to the ignition boxes. It is based on the Arduino Mega. A lesser microcontroller can be used, this was chosen due to availability and multiple UART ports. Having more than one will allow the serial bluetooth to stay connected during reprogramming.
The components of this box are
- Arduino Mega 2560
- 2-line display for debugging, status information
- Break-out board for connections and bluetooth adapter
- 12V lead-acid battery to power the controller box as well as all the ignition boxes
- RGB LED strip for status indication & fun
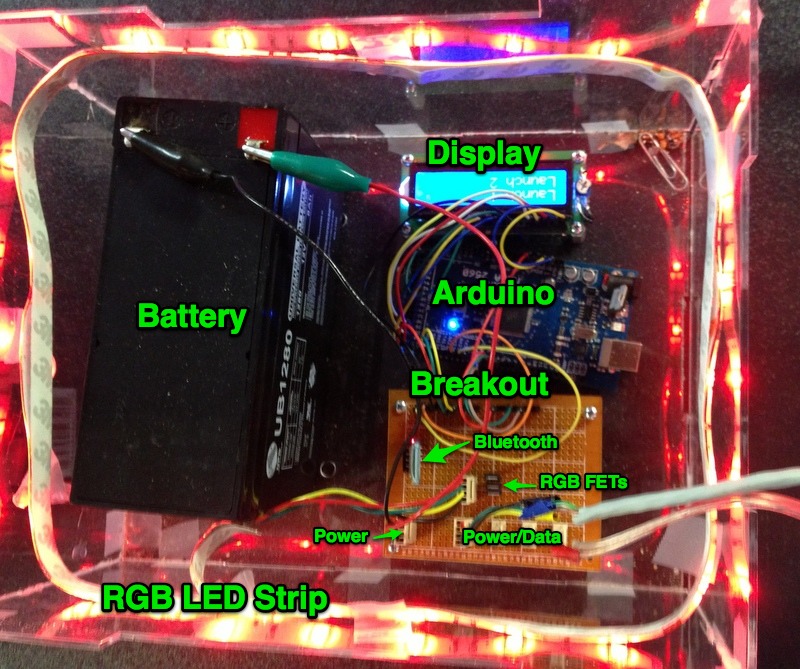
Code Overview
The Arduino Mega is programmed using the standard Arduino IDE with the Wire library to communicate to the ignition boxes and LiquidCrystal library for the display.
Setup configures the serial ports, LCD pin configurations, Wire configuration as a master device. In the main loop, read a character from the bluetooth serial port and check for a completed command ending in CR or LF characters. If a command is received, display it, scrolling up to display it. If it is a launch command, call the function to trigger the numbered igniter. Also monitor for a heartbeat and go offline if haven’t received a signal from the tablet in 4 seconds.
When a trigger command is received, the TriggerPin method is called. This will use the Wire library to send a command to the corresponding ignition box to activate the pin for 5 seconds.
Every second the status of every igniter is sent back to the tablet so it can display which outputs are active. A sequence of 100 characters are sent back with ‘0’ = off, ‘1’ = on or ‘2’ for unknown. The sequence is padded out to 100 if fewer igniter boxes are used. An additional code is sent for the controller status if any, and the whole sequence is enclosed in framing brackets ‘[]’.
The loop also handles color animations for the LED strip. The code currently starts up pulsing green, then flashes blue when a bluetooth connection is made. As long as there is a good connection, it will cycle red/white/blue colors. When any igniter is active, it will rapidly flash red and white.
Code Below
#include
#include
// LCD
LiquidCrystal lcd(22,28,29,27,25,23);
char line1[16];
char line2[16];
char line3[16];
int charpos = 0;
char welcome[] = "Ready";
// Serial Bluetooth
unsigned long timeLastSent = 0;
unsigned long timeLastReceived = 0;
boolean launchSequenceStarted = false;
int launchSequenceIndex = 0;
unsigned long timeLastSequence = 0;
// IC2
byte x[3];
boolean igniterOn[80];
enum cmds {
CMD_WRITE = 1,
CMD_WRITE_TIMED,
CMD_STOP_ALL,
CMD_READ_PINS
};
struct ic2cmd_write {
byte addr;
byte val;
};
struct ic2cmd_write_time {
byte addr;
int time;
};
// PWM LED Strip Vars
int redPin = 4;
int greenPin = 3;
int bluePin = 2;
float stripHue = 0;
float currentRed = 0;
float currentGreen = 0;
float currentBlue = 0;
int colorindex = 0;
unsigned long timeLastColor = 0;
int targetRed = 0;
int targetGreen = 0;
int targetBlue = 0;
float deltaRed = 0;
float deltaGreen = 0;
float deltaBlue = 0;
boolean isFading = false;
unsigned long timeLastFade;
int fadeInterval = 10;
enum LEDmode {
modeStart = 0,
modeConnect,
modeOnline,
modeLaunch
};
int ledMode = modeStart;
// LCD format for LCD display
String formatn(int val)
{
String ss = " ";
val = val % 100000;
int negadj = (val < 0) ? 1 : 0;
String sv = String(val);
String s = ss.substring(sv.length()) + sv;
return s;
}
// IC2 Send message to slave to activate igniter
void TriggerPin(int pin)
{
int slavenum = pin / 20;
ic2cmd_write_time sc;
sc.addr = pin % 20;
sc.time = 5000;
Wire.beginTransmission(4 + slavenum);
Wire.write(CMD_WRITE_TIMED);
Wire.write((byte*)&sc, sizeof(sc)); // sends one byte
Wire.endTransmission(); // stop transmitting
}
// IC2 send message to stop all igniters
void StopAll()
{
for (int s=0; s<5; s++)
{
Wire.beginTransmission(4 + s);
Wire.write(CMD_STOP_ALL);
Wire.endTransmission();
}
for (int i=0; i<80; i++) igniterOn[i] = false;
}
// LED Strip Red White Blue pattern
void patternRWB(float shift, float bright)
{
// shift = 0;
for (byte i=0; i<16; i++)
{
float dist = fmod(fabs(shift + i),16);
float cdist = dist / 2.0;
int lcolor = int(cdist);
int rcolor = (lcolor < 7) ? lcolor + 1 : 0;
float lweight = 1 - (cdist - lcolor);
float rweight = cdist - lcolor;
byte r = byte(min((rwbR[lcolor] * lweight + rwbR[rcolor] * rweight) * bright, 127.0));
byte g = byte(min((rwbG[lcolor] * lweight + rwbG[rcolor] * rweight) * bright, 127.0));
byte b = byte(min((rwbB[lcolor] * lweight + rwbB[rcolor] * rweight) * bright, 127.0));
colors[i * 3 + 1] = 0x80 | r;
colors[i * 3 + 0] = 0x80 | g;
colors[i * 3 + 2] = 0x80 | b;
}
for (int i = 0; i < 48; i++)
{
colors[48 + i] = colors[i];
}
}
void HSVtoRGB( float *r, float *g, float *b, float h, float s, float v )
{
int i;
float f, p, q, t;
if( s == 0 ) {
// achromatic (grey)
*r = *g = *b = v;
return;
}
h /= 60; // sector 0 to 5
i = floor( h );
f = h - i; // factorial part of h
p = v * ( 1 - s );
q = v * ( 1 - s * f );
t = v * ( 1 - s * ( 1 - f ) );
switch( i ) {
case 0:
*r = v;
*g = t;
*b = p;
break;
case 1:
*r = q;
*g = v;
*b = p;
break;
case 2:
*r = p;
*g = v;
*b = t;
break;
case 3:
*r = p;
*g = q;
*b = v;
break;
case 4:
*r = t;
*g = p;
*b = v;
break;
default: // case 5:
*r = v;
*g = p;
*b = q;
break;
}
}
// PWM LED strip color fade to set value
void fadeTo(int r, int g, int b, int t)
{
targetRed = r;
targetGreen = g;
targetBlue = b;
float dt = t / fadeInterval;
if (dt < 0.01) {
deltaRed = (r - currentRed);
deltaGreen = (g - currentGreen);
deltaBlue = (b - currentBlue);
} else {
deltaRed = (r - currentRed) / dt;
deltaGreen = (g - currentGreen) / dt;
deltaBlue = (b - currentBlue) / dt;
}
isFading = true;
}
void DoLEDFade()
{
if (isFading)
{
currentRed += deltaRed;
currentGreen += deltaGreen;
currentBlue += deltaBlue;
int red = currentRed;
int green = currentGreen;
int blue = currentBlue;
analogWrite(redPin, red);
analogWrite(greenPin, green);
analogWrite(bluePin, blue);
if (red == targetRed) deltaRed = 0;
if (green == targetGreen) deltaGreen = 0;
if (blue == targetBlue) deltaBlue = 0;
if ((red == targetRed) && (green == targetGreen) && (blue == targetBlue))
isFading = false;
}
}
void setup()
{
// PC Serial setup
Serial.begin(9600);
// bluetooth serial setup
Serial3.begin(9600);
// LCD setup
pinMode(24, OUTPUT);
digitalWrite(24, 0);
lcd.begin(16,2);
// clear buffers
memcpy(line1, 0, 16);
memcpy(line2, 0, 16);
memcpy(line3, 0, 16);
// print ready message
lcd.setCursor(0,0);
lcd.print(welcome);
// 2-Wire slave communication setup
Wire.begin(); // join i2c bus (address optional for master)
memset(x, 0, sizeof(x));
x[0] = 1;
x[1] = 1;
for (int i=0; i<80; i++) igniterOn[i] = false;
// SPI LED Strip setup
SPI.begin();
SPI.setClockDivider(SPI_CLOCK_DIV2);
SPI.setBitOrder(MSBFIRST);
rwbR[0] = 30; rwbG[0] = 0; rwbB[0] = 0;
rwbR[1] = 30; rwbG[1] = 0; rwbB[1] = 0;
rwbR[2] = 10; rwbG[2] = 10; rwbB[2] = 10;
rwbR[3] = 10; rwbG[3] = 10; rwbB[3] = 10;
rwbR[4] = 0; rwbG[4] = 0; rwbB[4] = 30;
rwbR[5] = 0; rwbG[5] = 0; rwbB[5] = 30;
rwbR[6] = 00; rwbG[6] = 00; rwbB[6] = 30;
rwbR[7] = 30; rwbG[7] = 00; rwbB[7] = 00;
patternRWB(0.0, 1.0);
}
void loop() {
unsigned long timenow = millis();
// Read input from bluetooth serial
if (Serial3.available()) {
char c = Serial3.read();
Serial.write(c); // echo to PC
if ((charpos == 16) || (c == 13)) {
timeLastReceived = timenow;
// if this is the 2sec heartbeat, throw away text reset row 3
if (strncmp(line3, "Online", 6) == 0)
{
for (int i = 0; i < 16; i++) {
line3[i] = 0;
charpos = 0;
}
} else {
// received end of line, shift line buffers
for (int i = 0; i < 16; i++) { line1[i] = line2[i]; line2[i] = line3[i]; line3[i] = 0; } // Refresh display lcd.setCursor(0,0); lcd.print(" "); lcd.setCursor(0,0); lcd.print(line1); lcd.setCursor(0,1); lcd.print(" "); lcd.setCursor(0,1); lcd.print(line2); charpos = 0; // Execute commands if (strncmp(line2, "Launch ", 7) == 0) { int index = atoi(&line2[7]); TriggerPin(index); ledMode = modeLaunch; colorindex = 0; /* // No launch squencing for 2013 // check for launch sequence start if (index == 76) { launchSequenceStarted = true; launchSequenceIndex = 76; timeLastSequence = timenow; } */ // Serial.write(line2); } else if (strncmp(line2, "Stop", 4) == 0) { // Serial.write("Stopping All\n"); StopAll(); } } } // Add received character to buffer if (c >= ' ' && c <= 'z') { line3[charpos] = c; charpos++; } } // check for sequencing if (launchSequenceStarted && (timenow - timeLastSequence > 20000))
{
timeLastSequence = timenow;
launchSequenceIndex++;
TriggerPin(launchSequenceIndex);
ledMode = modeLaunch;
colorindex = 0;
if (launchSequenceIndex == 80)
launchSequenceStarted = false;
}
// if we got a signal but in start mode switch to connect mode
if ((ledMode == modeStart) && (timeLastReceived > 0) && (timenow - timeLastReceived < 500)) { ledMode = modeConnect; colorindex = 0; } if ((ledMode == modeOnline) && (timenow - timeLastReceived > 4000))
{
ledMode = modeStart;
colorindex = 0;
}
/*
if (Serial.available())
Serial3.write(Serial.read());
*/
// lcd.setCursor(0,0);
// lcd.print(formatn(timenow));
// Once a second, get status from slaves and send over bluetooth
if ((timenow - timeLastSent) >= 1000)
{
// Serial.write("Checking Slaves\n\r");
// send updates once a second
timeLastSent = timenow;
Serial3.write("["); // framing value
Serial3.write("2"); // System code
for (int s = 0; s < 4; s++)
{
Wire.requestFrom(4 + s, 20);
byte nread = 0;
while (Wire.available())
{
byte b = Wire.read();
Serial3.write('0' + b);
nread++;
}
for (int i = nread; i < 20; i++) Serial3.write('2'); } Serial3.write("]\r\n"); // framing value } // PWM LED strip if (timenow - timeLastFade > fadeInterval)
{
DoLEDFade();
timeLastFade = timenow;
}
switch (ledMode) {
case modeStart:
{
if ((timenow - timeLastColor) > 2000)
{
timeLastColor = timenow;
colorindex++;
if (colorindex >= 2) colorindex = 0;
switch (colorindex)
{
case 0: fadeTo(0, 0, 0, 1600); break;
case 1: fadeTo(0, 50, 0, 1600); break;
}
}
} break;
case modeLaunch:
{
if ((timenow - timeLastColor) > 100)
{
timeLastColor = timenow;
switch (colorindex % 2)
{
case 0: fadeTo(80, 80, 80, 20); break;
case 1: fadeTo(120, 0, 0, 20); break;
}
colorindex++;
if (colorindex >= 30)
{
colorindex = 0;
ledMode = modeOnline;
}
}
} break;
case modeConnect:
{
if ((timenow - timeLastColor) > 300)
{
timeLastColor = timenow;
switch (colorindex)
{
case 0: fadeTo(0, 0, 100, 200); break;
case 1: fadeTo(0, 0, 0, 100); break;
case 2: fadeTo(0, 0, 100, 200); break;
case 3: fadeTo(0, 0, 0, 100); break;
}
colorindex++;
if (colorindex >= 3)
{
colorindex = 0;
ledMode = modeOnline;
}
}
} break;
case modeOnline:
{
if ((timenow - timeLastColor) > 3000)
{
timeLastColor = timenow;
colorindex++;
if (colorindex >= 3) colorindex = 0;
switch (colorindex)
{
case 0: fadeTo(100, 0, 0, 1500); break;
case 1: fadeTo(50, 50, 50, 1500); break;
case 2: fadeTo(0, 0, 100, 1500); break;
}
}
} break;
}
}